Customization & Changes
Change Language
1. Add Required Dependencies
Add the following dependencies in your pubspec.yaml file:
dependencies:
flutter_localizations:
sdk: flutter
intl: ^0.18.0
flutter_localizations:
sdk: flutter
intl: ^0.18.0
Run the command to fetch the packages:
flutter pub get
2. Create Translation Files
For each language, create separate JSON files in your assets folder (e.g., en.json for English and es.json for Spanish).
Directory Structure:
assets/
lang/
en.json
es.json
lang/
en.json
es.json
Example: assets/lang/en.json:
{
"title": "Welcome",
"greeting": "Hello, how are you?"
}
"title": "Welcome",
"greeting": "Hello, how are you?"
}
Example: assets/lang/es.json:
{
"title": "Bienvenido",
"greeting": "Hola, ¿cómo estás?"
}
"title": "Bienvenido",
"greeting": "Hola, ¿cómo estás?"
}
3. Load Translations
Create a helper class to manage translations.
Example: lib/l10n/app_localizations.dart:
import 'dart:convert';
import 'package:flutter/services.dart';
class AppLocalizations {
final Locale locale;
AppLocalizations(this.locale);
static AppLocalizations of(BuildContext context) {
return Localizations.of AppLocalizations(context, AppLocalizations)!;
}
static const LocalizationsDelegate AppLocalizations delegate = _AppLocalizationsDelegate();
Map String, String? _localizedStrings;
Future void load() async {
String jsonString = await rootBundle.loadString('assets/lang/${locale.languageCode}.json');
Map String, dynamic jsonMap = json.decode(jsonString);
_localizedStrings = jsonMap.map((key, value) => MapEntry(key, value.toString()));
}
String translate(String key) {
return _localizedStrings![key] ?? key;
}
}
class _AppLocalizationsDelegate extends LocalizationsDelegate AppLocalizations {
const _AppLocalizationsDelegate();
@override
bool isSupported(Locale locale) => ['en', 'es'].contains(locale.languageCode);
@override
Future AppLocalizations load(Locale locale) async {
AppLocalizations localizations = AppLocalizations(locale);
await localizations.load();
return localizations;
}
@override
bool shouldReload(covariant LocalizationsDelegate AppLocalizations old) => false;
}
import 'package:flutter/services.dart';
class AppLocalizations {
final Locale locale;
AppLocalizations(this.locale);
static AppLocalizations of(BuildContext context) {
return Localizations.of AppLocalizations(context, AppLocalizations)!;
}
static const LocalizationsDelegate AppLocalizations delegate = _AppLocalizationsDelegate();
Map String, String? _localizedStrings;
Future void load() async {
String jsonString = await rootBundle.loadString('assets/lang/${locale.languageCode}.json');
Map String, dynamic jsonMap = json.decode(jsonString);
_localizedStrings = jsonMap.map((key, value) => MapEntry(key, value.toString()));
}
String translate(String key) {
return _localizedStrings![key] ?? key;
}
}
class _AppLocalizationsDelegate extends LocalizationsDelegate AppLocalizations {
const _AppLocalizationsDelegate();
@override
bool isSupported(Locale locale) => ['en', 'es'].contains(locale.languageCode);
@override
Future AppLocalizations load(Locale locale) async {
AppLocalizations localizations = AppLocalizations(locale);
await localizations.load();
return localizations;
}
@override
bool shouldReload(covariant LocalizationsDelegate AppLocalizations old) => false;
}
4. Configure the App
Create a helper class to manage translations.
Update your MaterialApp to support localization.
Example: main.dart:
import 'package:flutter/material.dart';
import 'package:flutter_localizations/flutter_localizations.dart';
import 'l10n/app_localizations.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
class _MyAppState extends State {
Locale _locale = Locale('en');
void _changeLanguage(String languageCode) {
setState(() {
_locale = Locale(languageCode);
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
locale: _locale,
supportedLocales: [
Locale('en'), // English
Locale('es'), // Spanish
],
localizationsDelegates: [
AppLocalizations.delegate,
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
],
home: HomePage(onLanguageChanged: _changeLanguage),
);
}
}
class HomePage extends StatelessWidget {
final Function(String) onLanguageChanged;
const HomePage({required this.onLanguageChanged});
@override
Widget build(BuildContext context) {
var localizations = AppLocalizations.of(context);
return Scaffold(
appBar: AppBar(title: Text(localizations.translate('title'))),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(localizations.translate('greeting')),
SizedBox(height: 20),
ElevatedButton(
onPressed: () => onLanguageChanged('en'),
child: Text('English'),
),
ElevatedButton(
onPressed: () => onLanguageChanged('es'),
child: Text('Español'),
),
],
),
),
);
}
}
import 'package:flutter_localizations/flutter_localizations.dart';
import 'l10n/app_localizations.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
class _MyAppState extends State
Locale _locale = Locale('en');
void _changeLanguage(String languageCode) {
setState(() {
_locale = Locale(languageCode);
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
locale: _locale,
supportedLocales: [
Locale('en'), // English
Locale('es'), // Spanish
],
localizationsDelegates: [
AppLocalizations.delegate,
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
],
home: HomePage(onLanguageChanged: _changeLanguage),
);
}
}
class HomePage extends StatelessWidget {
final Function(String) onLanguageChanged;
const HomePage({required this.onLanguageChanged});
@override
Widget build(BuildContext context) {
var localizations = AppLocalizations.of(context);
return Scaffold(
appBar: AppBar(title: Text(localizations.translate('title'))),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(localizations.translate('greeting')),
SizedBox(height: 20),
ElevatedButton(
onPressed: () => onLanguageChanged('en'),
child: Text('English'),
),
ElevatedButton(
onPressed: () => onLanguageChanged('es'),
child: Text('Español'),
),
],
),
),
);
}
}
5. Add JSON Files to Assets
Add the JSON files to your pubspec.yaml file:
flutter:
assets:
- assets/lang/en.json
- assets/lang/es.json
assets:
- assets/lang/en.json
- assets/lang/es.json
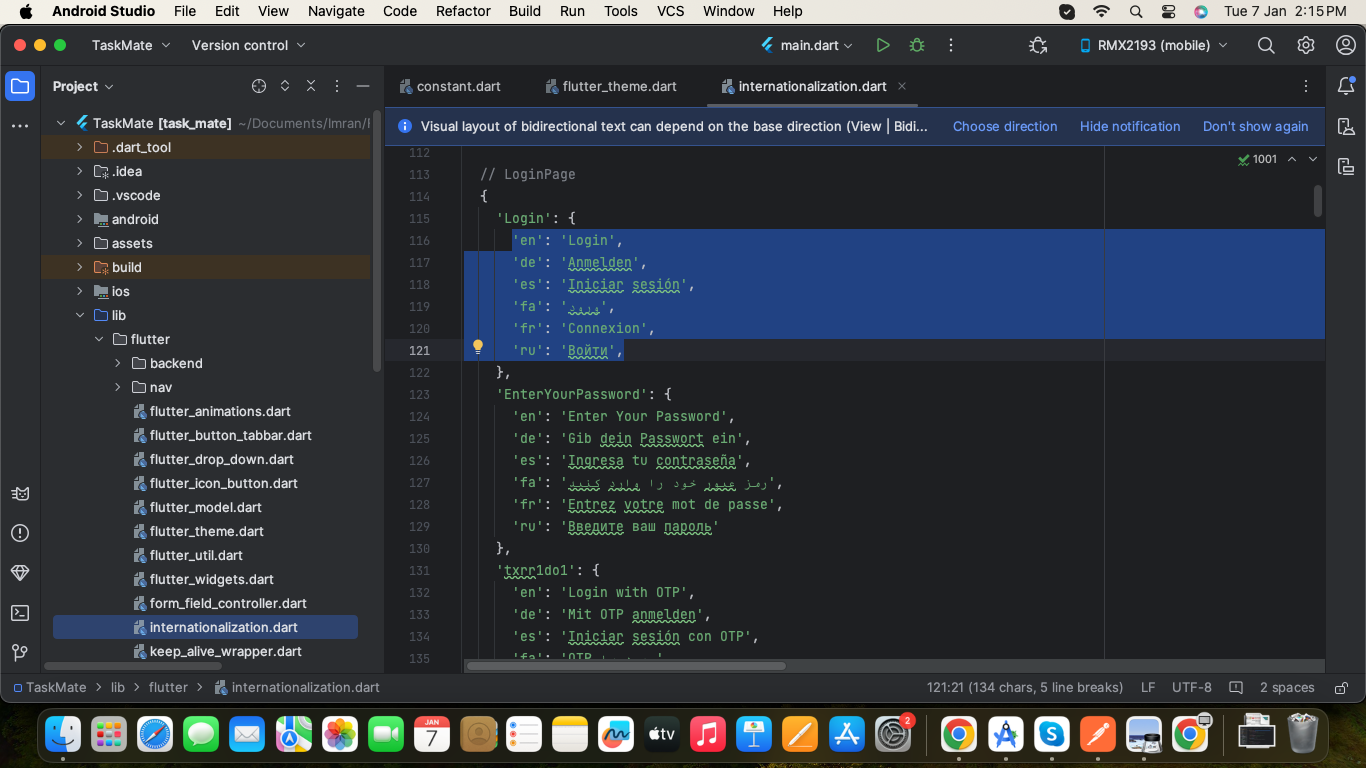
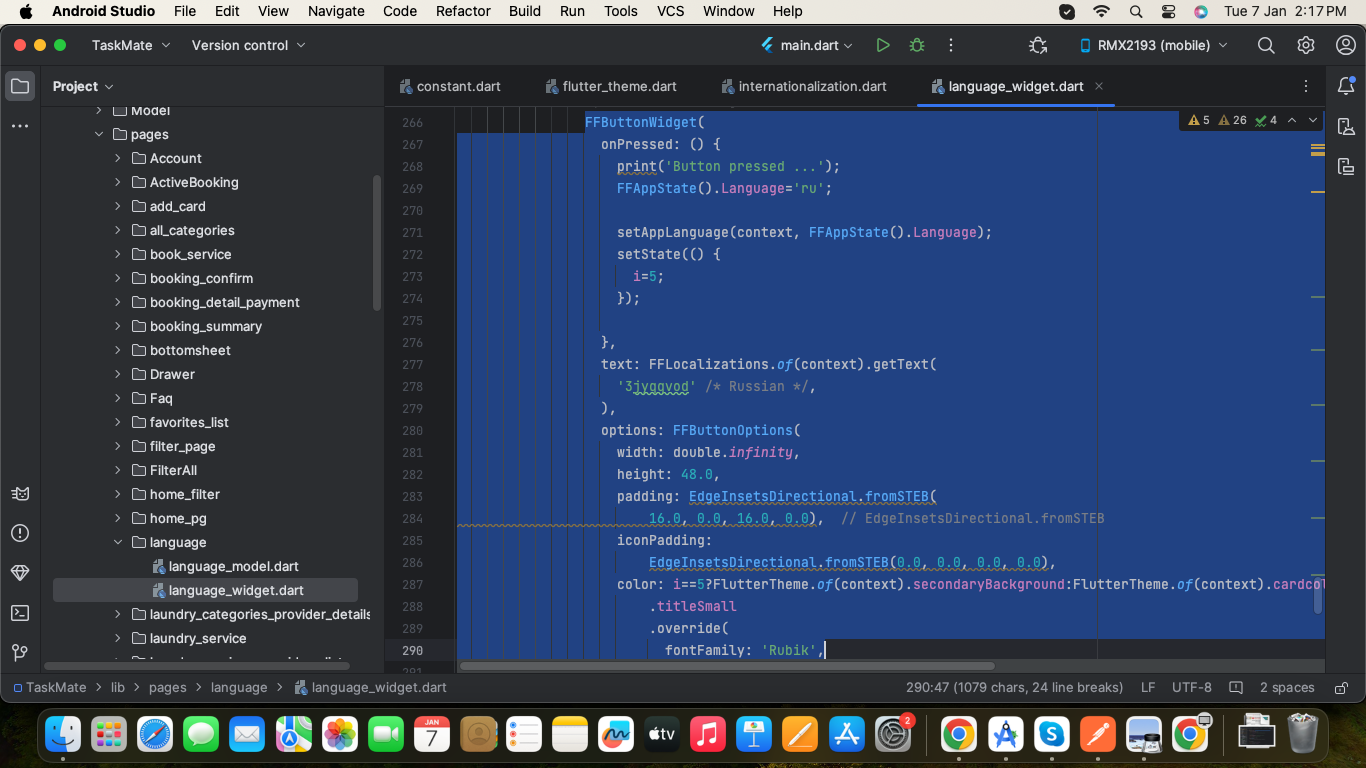