Customization & Changes
Change Stripe Payment Key
1. Locate the Stripe API Key Configuration
1. Check where the Stripe API keys are being used in your project. They are often stored in:
- A configuration file (e.g., config.dart, .env file).
- Directly in the code (though this is not recommended for security reasons).
- Environment variables.
2. Update the API Keys
Stripe provides two types of keys:
- Publishable Key: Used in the frontend (Flutter app) for client-side operations.
- Secret Key: Used in the backend for server-side operations.
Steps to Change Keys:
1. Find the Publishable Key in your Flutter project. Example:
const String stripePublishableKey = "your_old_publishable_key";
Replace it with the new key:
const String stripePublishableKey =
"your_new_publishable_key";
"your_new_publishable_key";
Update the Secret Key:
- The secret key should not be stored in the Flutter app for security reasons. Instead, it should be managed securely on your backend server.
- Update the secret key in your backend server configuration if needed.
3. Set the Key in Your Code
Ensure that the Stripe publishable key is set in your Flutter code. For example, if you're using the stripe_payment package:
import 'package:flutter_stripe/flutter_stripe.dart';
void main() {
Stripe.publishableKey = 'your_new_publishable_key';
runApp(MyApp()); }
void main() {
Stripe.publishableKey = 'your_new_publishable_key';
runApp(MyApp()); }
Or if using another package like stripe_payment:
StripePayment.setOptions(
StripeOptions(
publishableKey: "your_new_publishable_key",
merchantId: "your_merchant_id", // Optional
androidPayMode: "test", // Use "production" for live mode
),
);
StripeOptions(
publishableKey: "your_new_publishable_key",
merchantId: "your_merchant_id", // Optional
androidPayMode: "test", // Use "production" for live mode
),
);
4. Store Keys Securely
- Do not hard-code keys directly into your source code for security reasons.
- Use a secure method to load keys, such as:
1. Storing keys in environment variables using the flutter_dotenv package.
2. Fetching keys from a remote configuration service or backend.
Example with flutter_dotenv:
1. Add flutter_dotenv to your pubspec.yaml:
dependencies:
flutter_dotenv: ^5.0.2
flutter_dotenv: ^5.0.2
2. Create a .env file in your project root:
STRIPE_PUBLISHABLE_KEY=your_new_publishable_key
3. Load and use the key in your app:
import 'package:flutter_dotenv/flutter_dotenv.dart';
void main() async {
await dotenv.load();
Stripe.publishableKey = dotenv.env['STRIPE_PUBLISHABLE_KEY']!;
runApp(MyApp());
}
void main() async {
await dotenv.load();
Stripe.publishableKey = dotenv.env['STRIPE_PUBLISHABLE_KEY']!;
runApp(MyApp());
}
5. Test the Integration
- For Test Mode: Use test keys from your Stripe Dashboard and test with Stripe’s test cards.
- For Live Mode: Replace test keys with live keys and perform a live transaction.
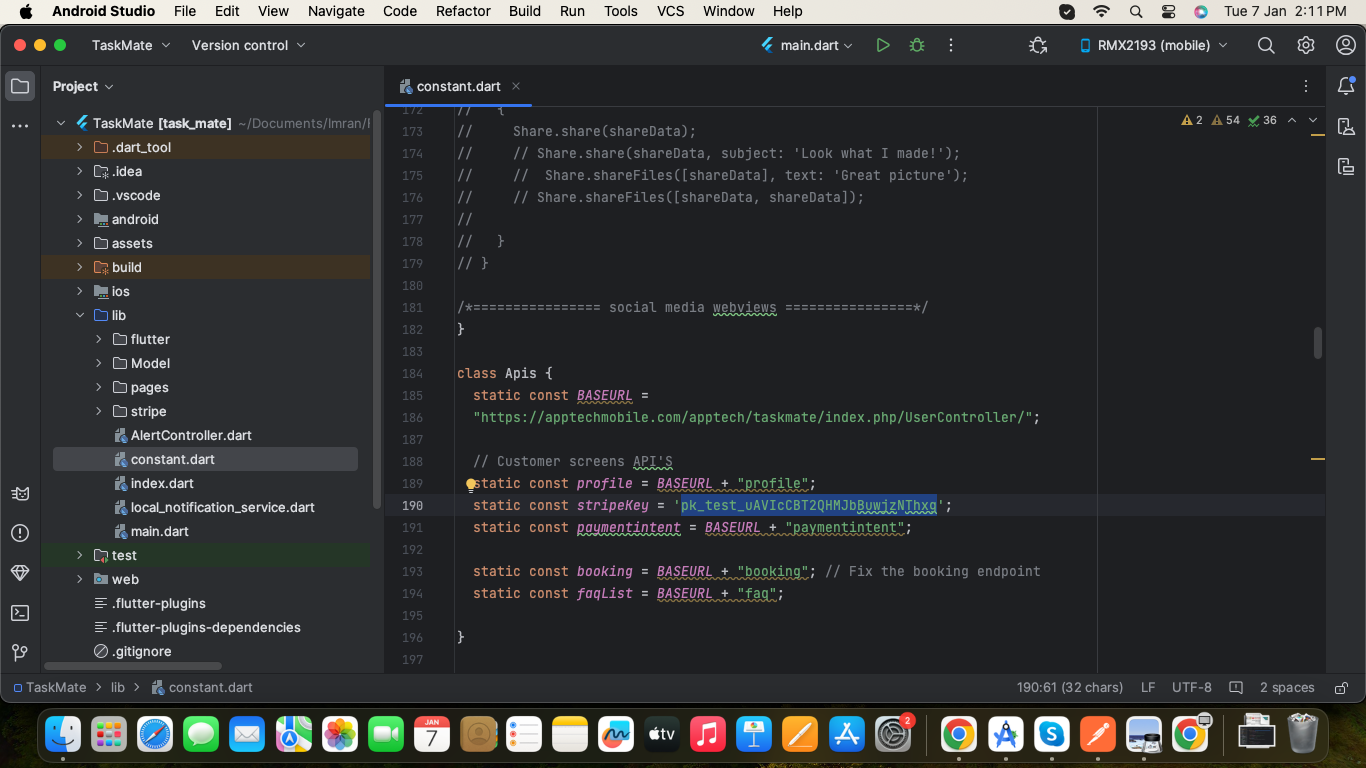