Customization & Changes
Change Base Url
1. Locate the Configuration File
If your project uses a dedicated file for managing constants, locate it. Typically, this is a constants.dart file or similar.
// lib/constants.dart
class AppConfig {
static const String baseUrl = "https://api.example.com/";
}
class AppConfig {
static const String baseUrl = "https://api.example.com/";
}
2. Update the Base URL
Modify the baseUrl constant in the configuration file to the desired URL.
// lib/constants.dart
class AppConfig {
static const String baseUrl = "https://api.newexample.com/";
}
class AppConfig {
static const String baseUrl = "https://api.newexample.com/";
}
3. Using Environment-Specific Base URLs
For environment-specific configurations (e.g., dev, staging, production), you can use flavor management or Dart's environment variables.
Option 1: Using a config file
Create separate config files for each environment.
lib/configs/dev_config.dart:
class AppConfig {
static const String baseUrl = "https://dev.api.example.com/";
}
static const String baseUrl = "https://dev.api.example.com/";
}
lib/configs/prod_config.dart:
class AppConfig {
static const String baseUrl = "https://api.example.com/";
}
static const String baseUrl = "https://api.example.com/";
}
Switch the imported file based on the environment.
4. Update the API Call
Ensure the base URL is used in your HTTP requests.
Example
import 'package:http/http.dart' as http;
import 'constants.dart';
Future void fetchData() async {
final response = await http.get(Uri.parse('${AppConfig.baseUrl}endpoint'));
if (response.statusCode == 200) {
print('Data: ${response.body}');
} else {
print('Error: ${response.statusCode}');
}
}
import 'constants.dart';
Future void fetchData() async {
final response = await http.get(Uri.parse('${AppConfig.baseUrl}endpoint'));
if (response.statusCode == 200) {
print('Data: ${response.body}');
} else {
print('Error: ${response.statusCode}');
}
}
5. Using Flutter's --dart-define for Environment Variables
You can dynamically set the base URL using the --dart-define flag.
Step 1: Update the code to use environment variables
class AppConfig {
static String get baseUrl => const String.fromEnvironment('BASE_URL', defaultValue: 'https://default.api.com/');
}
static String get baseUrl => const String.fromEnvironment('BASE_URL', defaultValue: 'https://default.api.com/');
}
Step 2: Pass the environment variable during build or run
For development:
flutter run --dart-define=BASE_URL=https://dev.api.example.com/
For release:
flutter build apk
--dart-define=BASE_URL=https://prod.api.example.com/
--dart-define=BASE_URL=https://prod.api.example.com/
6. Rebuild the App
Rebuild the app to apply the new base URL:
flutter clean
flutter pub get
flutter run
flutter pub get
flutter run
7. Verify the Configuration
Test the app to ensure API calls are directed to the new base URL.
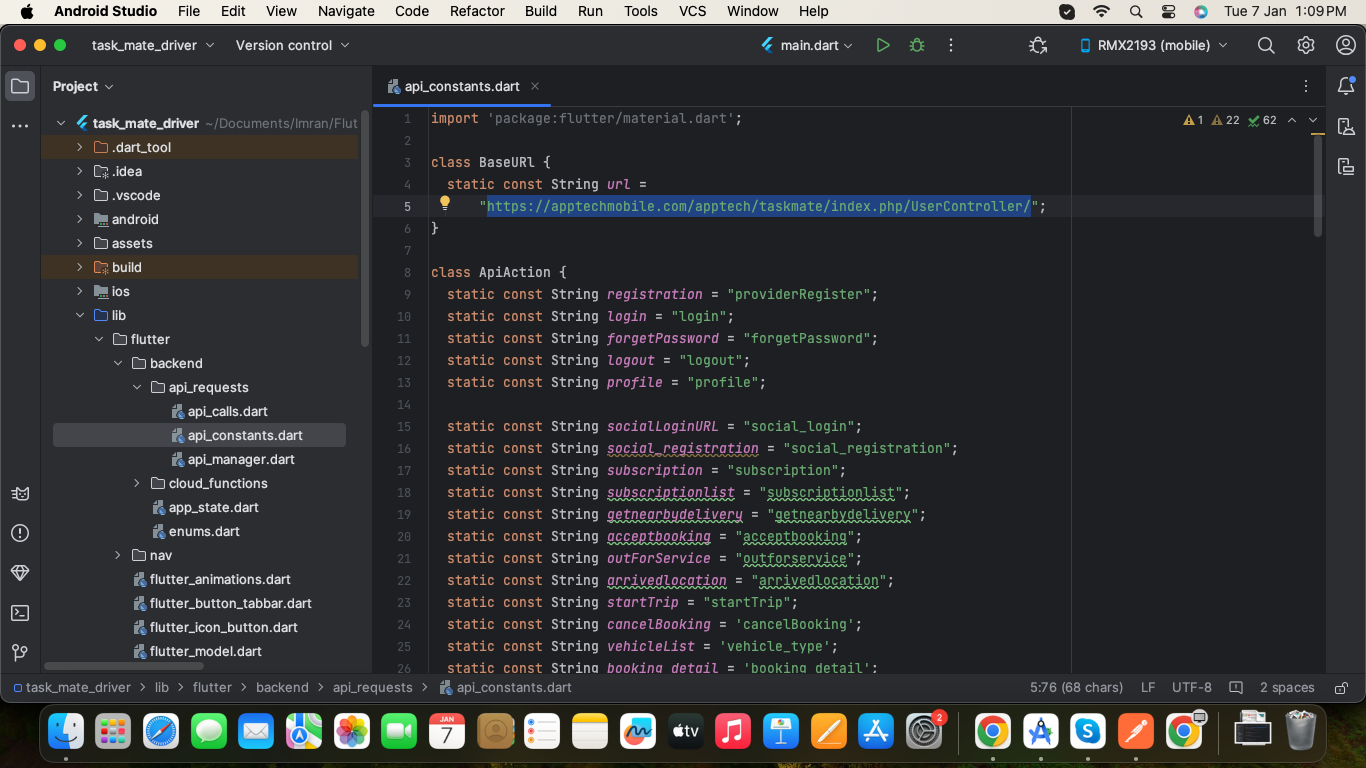