Customization & Changes
Change App Theme & Color
1. Changing Theme Colors
You can define a theme in your app's MaterialApp widget. This is the preferred way to manage colors consistently across your app.
MaterialApp(
theme: ThemeData(
primaryColor: Colors.blue, // Sets the primary color
accentColor: Colors.amber, // Sets the accent color
scaffoldBackgroundColor: Colors.grey[100], // Sets the background color
),
home: MyHomePage(),
);
theme: ThemeData(
primaryColor: Colors.blue, // Sets the primary color
accentColor: Colors.amber, // Sets the accent color
scaffoldBackgroundColor: Colors.grey[100], // Sets the background color
),
home: MyHomePage(),
);
2. Using Colors Directly in Widgets
You can specify colors for individual widgets.
Container(
color: Colors.red, // Set the container's background color
child: Text(
'Hello, Flutter!',
style: TextStyle(color: Colors.white), // Set the text color
),
),
color: Colors.red, // Set the container's background color
child: Text(
'Hello, Flutter!',
style: TextStyle(color: Colors.white), // Set the text color
),
),
3. Using Custom Colors
You can create a custom color palette in a separate Dart file for better organization.
Example: Define a colors.dart file
import 'package:flutter/material.dart';
class AppColors {
static const Color primary = Color(0xFF0A73EC);
static const Color secondary = Color(0xFFFFA500);
static const Color background = Color(0xFFF5F5F5);
}
class AppColors {
static const Color primary = Color(0xFF0A73EC);
static const Color secondary = Color(0xFFFFA500);
static const Color background = Color(0xFFF5F5F5);
}
Use the custom colors
Container(
color: AppColors.primary,
child: Text(
'Custom Color',
style: TextStyle(color: AppColors.secondary),
),
),
color: AppColors.primary,
child: Text(
'Custom Color',
style: TextStyle(color: AppColors.secondary),
),
),
4. Using Gradient Colors
If you want to apply gradients, you can use the BoxDecoration in a container.
Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blue, Colors.purple],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
),
child: Center(
child: Text(
'Gradient Background',
style: TextStyle(color: Colors.white),
),
),
),
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blue, Colors.purple],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
),
child: Center(
child: Text(
'Gradient Background',
style: TextStyle(color: Colors.white),
),
),
),
5. Dynamically Changing Colors
For dynamic changes, you can use state management solutions like setState, Provider, or Riverpod.
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State MyApp {
Color backgroundColor = Colors.white;
void changeColor() {
setState(() {
backgroundColor = Colors.lightBlue;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: backgroundColor,
appBar: AppBar(title: Text('Dynamic Colors')),
body: Center(
child: ElevatedButton(
onPressed: changeColor,
child: Text('Change Color'),
),
),
);
}
}
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State MyApp {
Color backgroundColor = Colors.white;
void changeColor() {
setState(() {
backgroundColor = Colors.lightBlue;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: backgroundColor,
appBar: AppBar(title: Text('Dynamic Colors')),
body: Center(
child: ElevatedButton(
onPressed: changeColor,
child: Text('Change Color'),
),
),
);
}
}
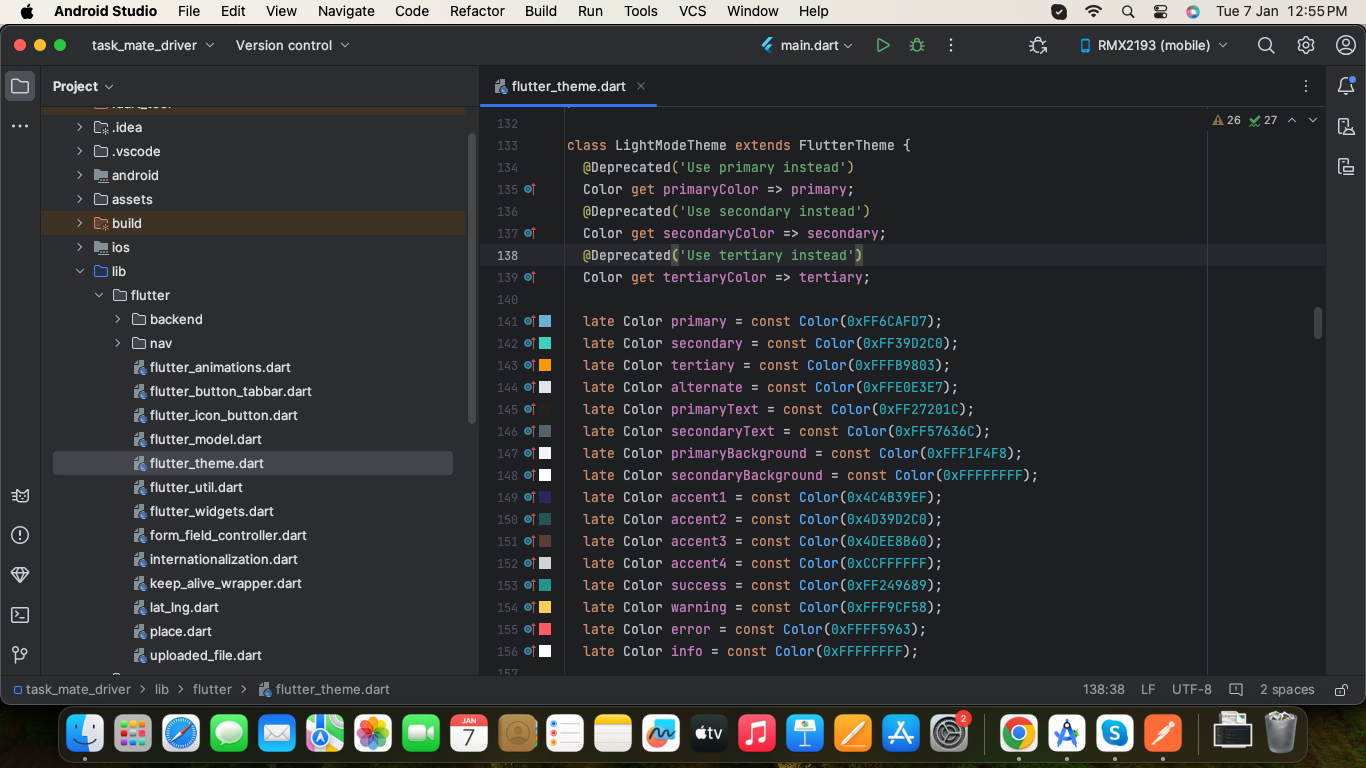