Customization & Changes
Change Google Mapkey
1. Locate the API Key
In your Flutter project, the Google Maps API Key is typically stored in the following files:
For Android:
- The API key is defined in the
android/app/src/main/AndroidManifest.xml file under the
application tag
For iOS:
- The API key is defined in the
ios/Runner/AppDelegate.swift file or the
ios/Runner/AppDelegate.m file, depending on whether you're using Swift or Objective-C
2. Obtain a New API Key
If you don't already have a key, generate one:
1. Go to the Google Cloud Console.
2. Create a new project or select an existing one.
3. Navigate to APIs & Services > Credentials.
4. Click Create Credentials > API Key
5. Copy the generated API key.
3. Update the API Key in Flutter
For Android:
1. Open android/app/src/main/AndroidManifest.xml.
2. Locate the following block inside the application tag:
meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_OLD_API_KEY" />
3.Replace YOUR_OLD_API_KEY with your new key:
meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_NEW_API_KEY" />
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_OLD_API_KEY" />
3.Replace YOUR_OLD_API_KEY with your new key:
meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_NEW_API_KEY" />
For iOS:
1. Open ios/Runner/AppDelegate.swift (or AppDelegate.m for Objective-C).
2. Locate the line where the API key is set:
GMSServices.provideAPIKey("YOUR_OLD_API_KEY")
3. Replace YOUR_OLD_API_KEY with your new key:
GMSServices.provideAPIKey("YOUR_NEW_API_KEY")
4. Update Key in google_maps_flutter Plugin (if applicable)
If you're using the google_maps_flutter plugin and initializing the key in your Flutter code, you may need to update it there as well.
Example:
GoogleMap(
initialCameraPosition: CameraPosition(
target: LatLng(37.7749, -122.4194),
zoom: 10,
),
apiKey: "YOUR_NEW_API_KEY", // Update the key here, if explicitly defined
);
initialCameraPosition: CameraPosition(
target: LatLng(37.7749, -122.4194),
zoom: 10,
),
apiKey: "YOUR_NEW_API_KEY", // Update the key here, if explicitly defined
);
5. Enable Required APIs
Ensure the following APIs are enabled in the Google Cloud Console:
- Maps SDK for Android
- Maps SDK for iOS
- Places API (if using place searches)
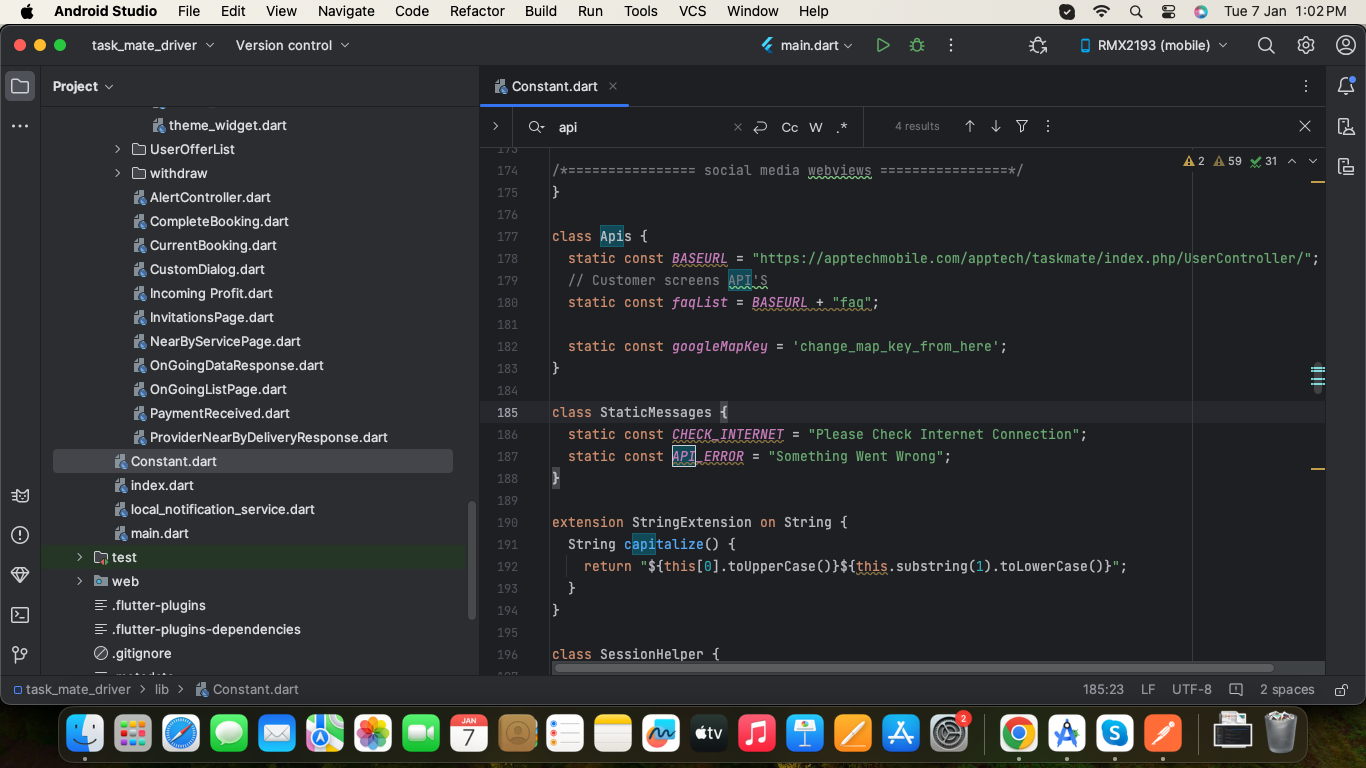
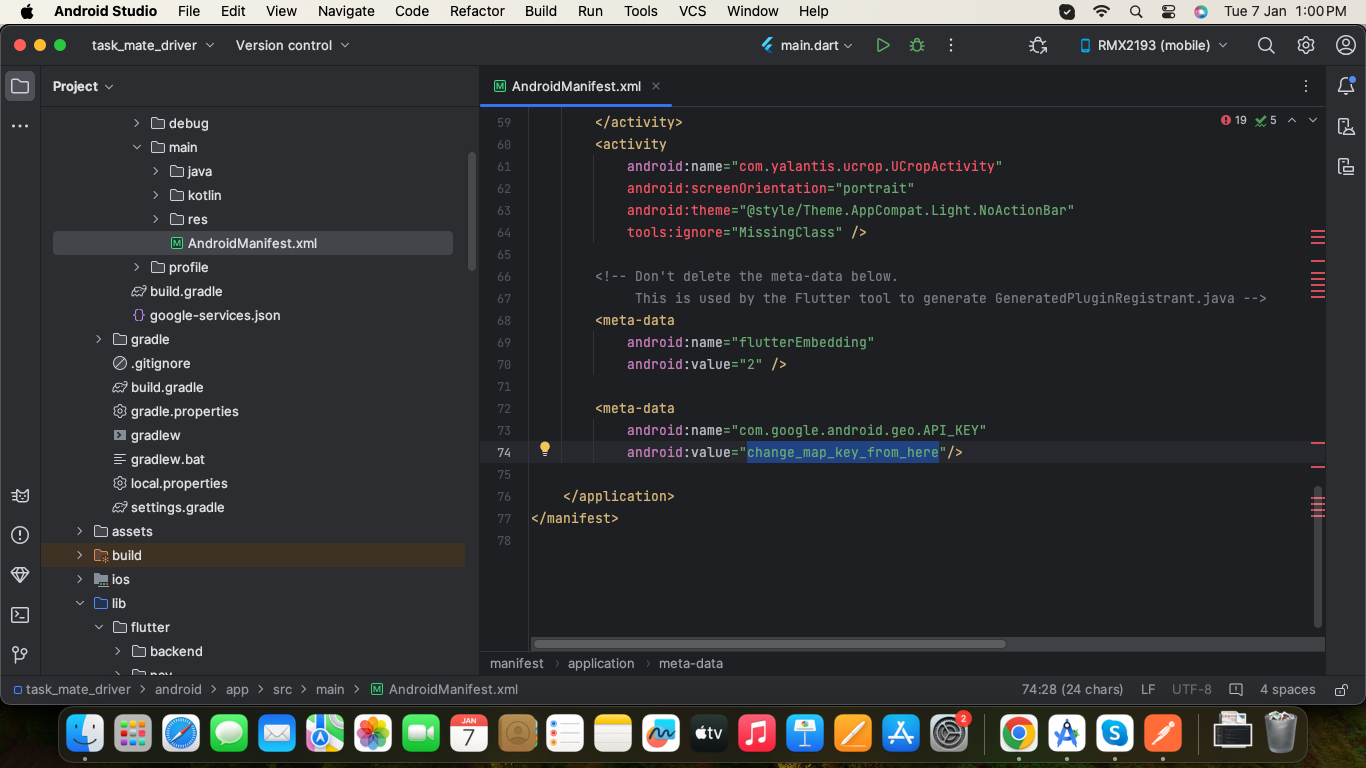